# core.customizeAllInstances
Extend all definition instances viewer capabilities by implement a range of customizations, such as controlling the instance's display, determining field visibility, adjusting box sizes, and more.
# Usage:
core.customizeAllInstances(function(instance, presenter) {
...
})
Arguments:
Argument | Description | Required |
---|---|---|
function | Callback function responsible to implement the new functionality | YES |
Function arguments:
Argument | Description |
---|---|
instance | The instance model. |
presenter | The instance presenter |
# Examples:
In the code snippet below, we'll present a file preview exclusively for image files
cob.custom.customize.push(function(core, utils, ui) {
core.customizeAllInstances(function(instance, presenter) {
const supportedExtensions = /\.(gif|jpg|png|webp)/i;
const questionB64 = "data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIGZpbGw9Im5vbmUiIHZpZXdCb3g9IjAgMCAyNCAyNCIgc3Ryb2tlLXdpZHRoPSIxLjUiIHN0cm9rZT0iY3VycmVudENvbG9yIiBjbGFzcz0idy02IGgtNiI+CiAgICA8cGF0aCBzdHJva2UtbGluZWNhcD0icm91bmQiIHN0cm9rZS1saW5lam9pbj0icm91bmQiIGQ9Ik05Ljg3OSA3LjUxOWMxLjE3MS0xLjAyNSAzLjA3MS0xLjAyNSA0LjI0MiAwIDEuMTcyIDEuMDI1IDEuMTcyIDIuNjg3IDAgMy43MTItLjIwMy4xNzktLjQzLjMyNi0uNjcuNDQyLS43NDUuMzYxLTEuNDUuOTk5LTEuNDUgMS44Mjd2Ljc1TTIxIDEyYTkgOSAwIDEgMS0xOCAwIDkgOSAwIDAgMSAxOCAwWm0tOSA1LjI1aC4wMDh2LjAwOEgxMnYtLjAwOFoiIC8+Cjwvc3ZnPgo=";
const classImgPreview = "js-img-preview";
/**
* Add dom element that allows to preview an image
* @param instance the instance model
* @param fp the field presenter
*/
function showImagePreview(instance, fp) {
const fpHtml = fp.content()[0];
// remove previous preview if existing
const previousPreview = fpHtml.querySelector(`.${classImgPreview}`);
if (previousPreview) previousPreview.remove();
// add new preview pointing to the image
if (fp.getValue()) {
const url = `/recordm/recordm/instances/${instance.getId()}/files/${fp.field.fieldDefinition.id}/${fp.getValue()}`;
fpHtml.insertAdjacentHTML("beforeend",
supportedExtensions.test(fp.getValue())
? `<div class="${classImgPreview} mt-2"><img src="${url}" alt="picture non-editable" style="max-width: 200px;"></div>`
: `<div class="${classImgPreview} mt-2"><img src="${questionB64}" alt="unknown" width="50"></div>`,
);
}
}
/**
* Extract all fields from the presenter that are of type $file and show the preview
*/
presenter
.findFieldPs((fp) => /\$file/.test(fp.field.fieldDefinition.description) && fp.getValue() !== null)
.forEach(fp => {
showImagePreview(instance, fp);
presenter.onFieldChange(fp, () => showImagePreview(instance, fp));
});
});
});
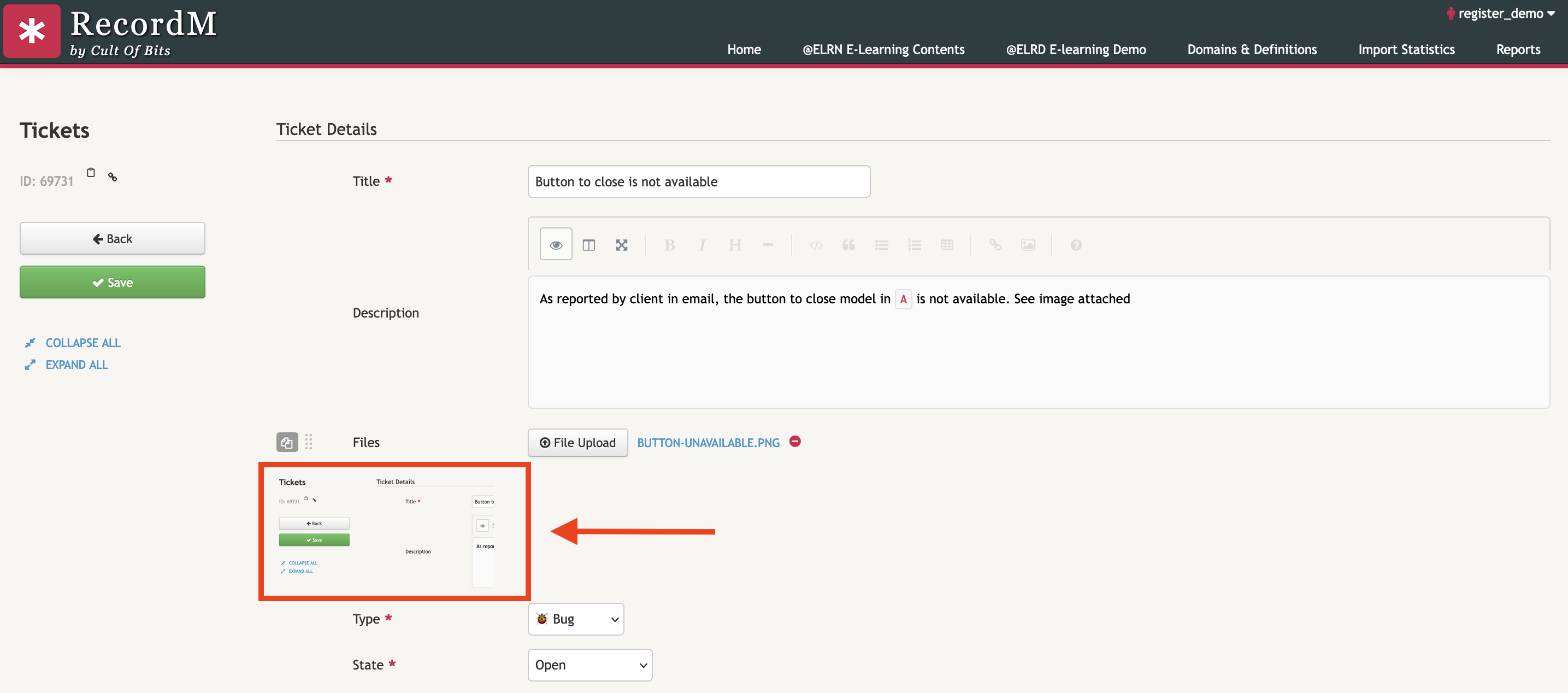