# core.customizeListing
Enhance a definition's search results by enriching the UI with additional information, buttons, and insights.
# Usage:
core.customizeListing(<definition-name>, {
onSelection: function(listingContainer, results){
...
},
onResults: function(listingContainer, results){
...
},
})
Arguments:
Argument | Description | Required |
---|---|---|
definition-name | Refers to the name of the specific definition to which you want to apply the customization. | YES |
function | Callback function responsible to implement the custom action of an instance. Possible functions are onSelection or onResults that run, respectively, when instances are selected and when they are show per search. | YES |
Function arguments:
Argument | Description |
---|---|
listingContainer | The DOM container for the entire listing. |
results | The listing results - a list of the shown instances. |
# Examples:
A common use for this customization is for definitions centered around number values or "easily accessible counters". In the following example, we go over all the visible Tickets to count them according to their state. We then allow the user to quickly view them by presenting the results on top of the listing.
// Adiciona totais seleccionados à listagem
cob.custom.customize.push(function (core, utils, ui) {
const DEFINITION = "Tickets";
// Open, Waiting For info, Done, Canceled
core.customizeListing(DEFINITION, {
onResults: async function (listingContainer, results) {
let insertionPoint = listingContainer.getElementsByClassName('left-container')[0];
let counters = { "Open": 0, "Waiting For info": 0, "Done": 0, "Canceled": 0 }
if (document.getElementById('js-mytotals') === null) {
insertionPoint.insertAdjacentHTML('beforeend', '<div id="js-mytotals" class="pt-2"></div>');
}
results.map((r) => {
switch (r['state'][0]) {
case "Open": counters['Open']++; break;
case "Waiting For info": counters['Waiting For info']++; break;
case "Done": counters['Done']++; break;
case "Canceled": counters['Canceled']++; break;
}
})
document.getElementById("js-mytotals").
innerHTML = (`Total Tickets (${results.length}) | Open: <span class='bg-red-100 text-red-800 text-xs font-medium me-2 px-2.5 py-0.5 rounded-full dark:bg-red-900 dark:text-red-300'>${counters['Open']}</span> `
+ `| Waiting: <span class='bg-yellow-100 text-yellow-800 text-xs font-medium me-2 px-2.5 py-0.5 rounded-full dark:bg-yellow-900 dark:text-yellow-300'>${counters['Waiting For info']}</span> `
+ `| Done: <span class='bg-green-100 text-green-800 text-xs font-medium me-2 px-2.5 py-0.5 rounded-full dark:bg-green-900 dark:text-green-300'>${counters['Done']}</span> `
+ `| Canceled: <span class='font-bold'>${counters['Canceled']}</span> `
)
}
})
});
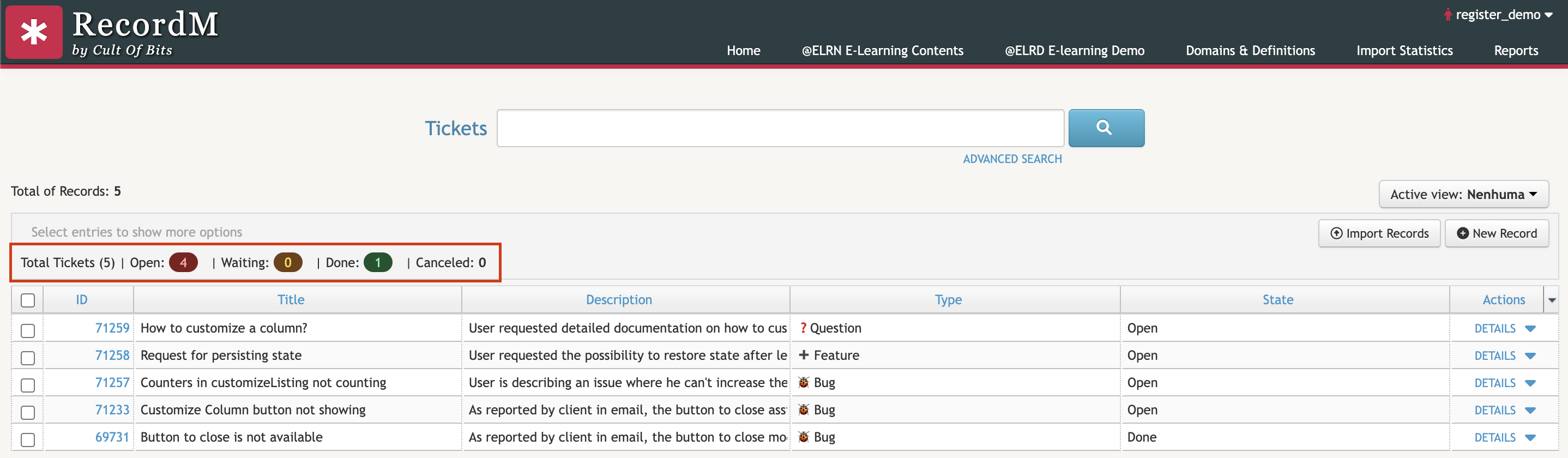